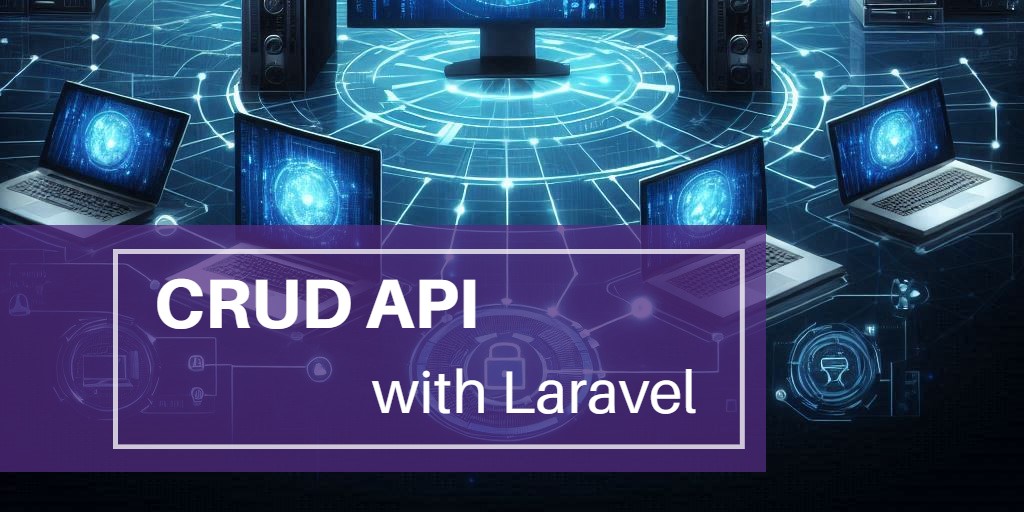
Create a CRUD API with Laravel
The CRUD operations (create, read, update, delete) are the basic functionality of any web application when working with a database. This example will show you how to create the CRUD API with Laravel and use MySQL as a database.
Prerequisites
- Composer
- PHP 8.2
- MySQL
Setup project
composer create-project laravel/laravel laravel_api 11.0.3
Create a testing database named "example" and run the database.sql file to import the table and data.
Project structure
├─ .env
├─ app
│ ├─ Http
│ │ └─ Controllers
│ │ └─ ProductController.php
│ └─ Models
│ └─ Product.php
├─ bootstrap
│ └─ app.php
├─ resources
│ └─ views
│ └─ index.php
└─ routes
├─ api.php
└─ web.php
*This project structure will show only files and folders that we intend to create or modify.
Project files
.env
This file is the Laravel configuration file and we use it to keep the database connection information.
DB_CONNECTION=mysql
DB_HOST=localhost
DB_PORT=3306
DB_DATABASE=example
DB_USERNAME=root
DB_PASSWORD=
SESSION_DRIVER=file
We also set SESSION_DRIVER=file
to change the session driver from database to file.
app.php
This file is the Laravel application configuration file, and we only added the API routing file here.
<?php
use Illuminate\Foundation\Application;
use Illuminate\Foundation\Configuration\Exceptions;
use Illuminate\Foundation\Configuration\Middleware;
return Application::configure(basePath: dirname(__DIR__))
->withRouting(
web: __DIR__.'/../routes/web.php',
api: __DIR__.'/../routes/api.php',
commands: __DIR__.'/../routes/console.php',
health: '/up',
)
->withMiddleware(function (Middleware $middleware) {
//
})
->withExceptions(function (Exceptions $exceptions) {
//
})->create();
web.php
This file defines the route URL for the Laravel web application. We just changed the default file from welcome.php to index.php.
<?php
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return view('index');
});
api.php
This file defines the route URL for the Laravel API. We define our API route here.
<?php
use App\Http\Controllers\ProductController;
Route::resource('/products', ProductController::class);
Product.php
This file defines the Eloquent Model information that maps to our database table named "Product".
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
protected $table = 'Product';
protected $primaryKey = 'id';
protected $fillable = [ 'name', 'price' ];
public $timestamps = false;
}
$fillable = [ 'name', 'price' ]
the column list that allows you to insert and update.$timestamps = false
does not utilize the auto-generate timestamp feature (create_at, update_at columns).
ProductController.php
This file defines all functions required to handle incoming requests and perform any CRUD operations.
<?php
namespace App\Http\Controllers;
use App\Models\Product;
class ProductController {
public function index()
{
return Product::get();
}
public function show($id)
{
return Product::find($id);
}
public function store()
{
return Product::create([
'name' => request()->input('name'),
'price' => request()->input('price')
]);
}
public function update($id)
{
return tap(Product::find($id))->update([
'name' => request()->input('name'),
'price' => request()->input('price')
]);
}
public function destroy($id)
{
Product::find($id)->delete();
}
}
We use an Eloquent model named Product to perform any CRUD operations on the database by using the basic methods such as get, find, create, update, delete
index.php
This file will be used to create a basic user interface for testing our API.
<!DOCTYPE html>
<head>
<style>
li {
margin-bottom: 5px;
}
textarea {
width: 100%;
}
</style>
</head>
<body>
<h1>Example CRUD</h1>
<ul>
<li><button onclick="getProducts()">Get Products</button></li>
<li><button onclick="getProduct()">Get Product</button></li>
<li><button onclick="createProduct()">Create Product</button></li>
<li><button onclick="updateProduct()">Update Product</button></li>
<li><button onclick="deleteProduct()">Delete Product</button></li>
</ul>
<textarea id="text_response" rows="20"></textarea>
<script>
function showResponse(res) {
res.text().then(text => {
let contentType = res.headers.get('content-type')
if (contentType && contentType.startsWith('application/json')) {
text = JSON.stringify(JSON.parse(text), null, 4)
}
document.getElementById('text_response').innerHTML = text
})
}
function getProducts() {
fetch('/api/products').then(showResponse)
}
function getProduct() {
let id = prompt('Input product id')
fetch('/api/products/' + id).then(showResponse)
}
function createProduct() {
let name = prompt('Input product name')
let price = prompt('Input product price')
fetch('/api/products', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name, price })
}).then(showResponse)
}
function updateProduct() {
let id = prompt('Input product id to update')
let name = prompt('Input new product name')
let price = prompt('Input new product price')
fetch('/api/products/' + id, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name, price })
}).then(showResponse)
}
function deleteProduct() {
let id = prompt('Input product id to delete')
fetch('/api/products/' + id, {
method: 'DELETE'
}).then(showResponse)
}
</script>
</body>
</html>
- Many other articles will use Postman as the HTTP client to test the API, but in this article, I will use JavaScript instead. This will help you understand more details when working with HTTP request on the client-side.
- To keep this file clean and readable, we will only use basic HTML and JavaScript. There are no additional libraries such as the CSS Framework or Axios here.
- All CRUD functions will use the appropriate HTTP method to invoke the API.
showResponse(res)
will format the JSON object to make it easier to read.
Run project
php artisan serve
Open the web browser and goto http://localhost:8000
Testing
Get All Products
Click the "Get Products" button. The API will return all product data.
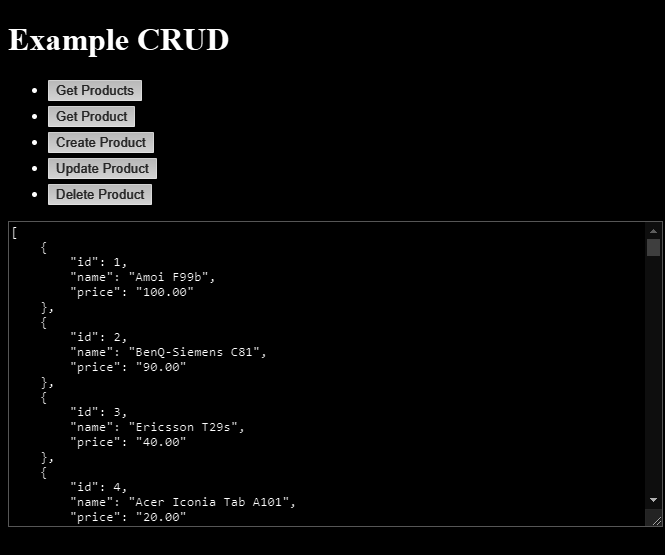
Get Product By Id
Click the "Get Product" button and enter "1" for the product id. The API will return a product data.
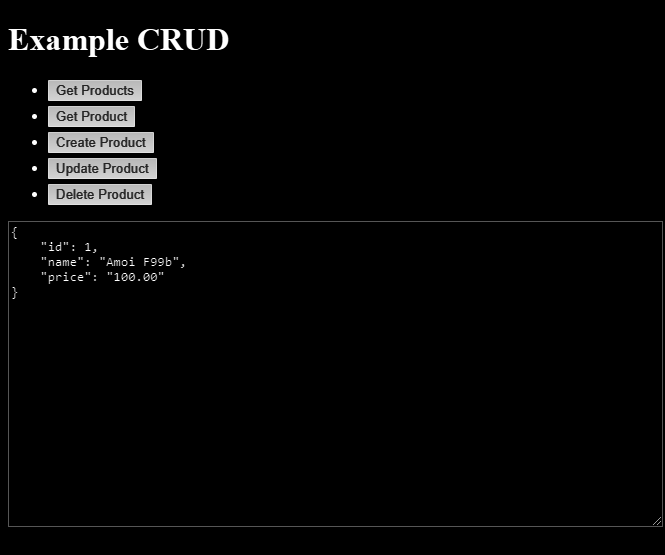
Create Product
Click the "Create Product" button and enter "test-create" for the product name and "100" for the price. The API will return a newly created product.
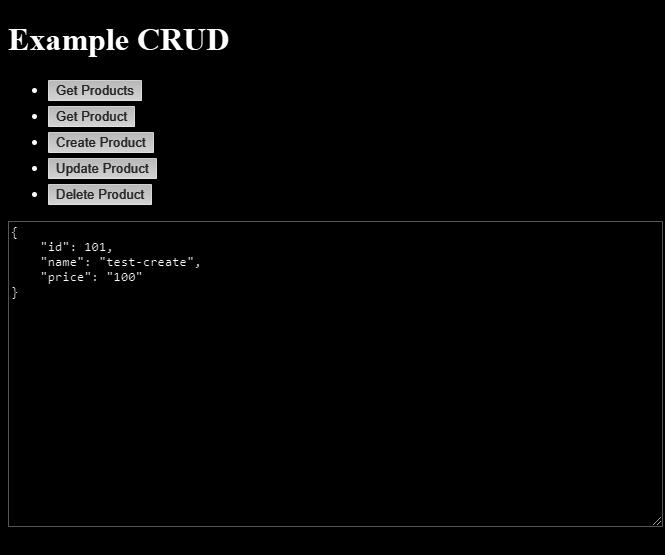
Update Product
Click the "Update Product" button and enter "101" for the product id and "test-update" for the name and "200" for the price. The API will return an updated product.
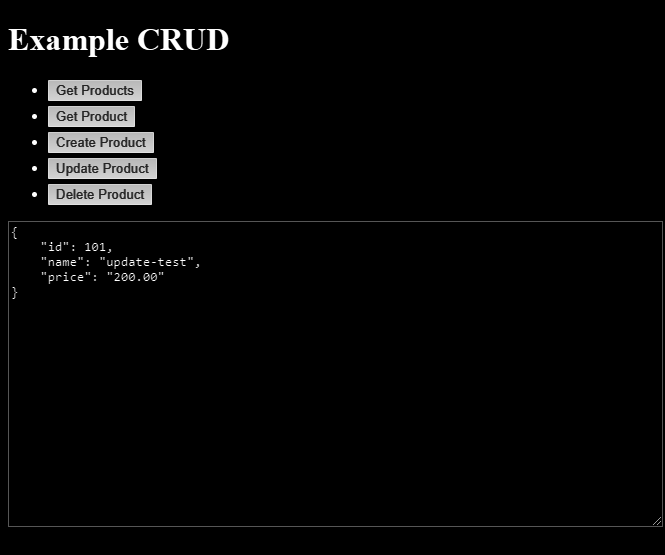
Delete Product
Click the "Delete Product" button and enter "101" for the product id. The API will return nothing, which is acceptable as we do not return anything from our API.
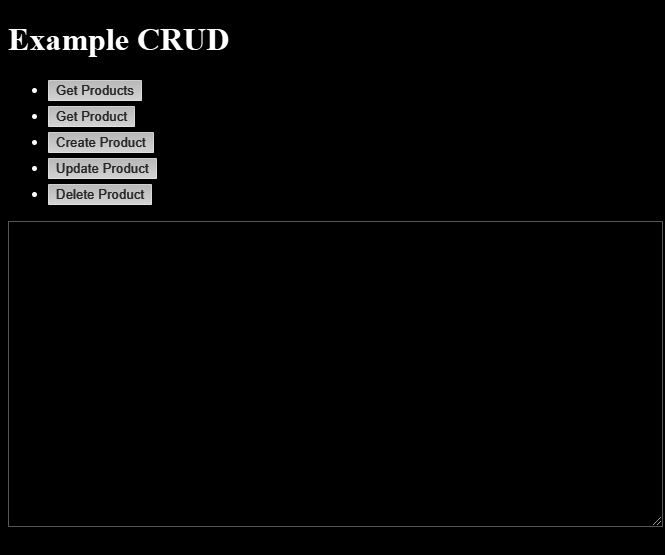
Conclusion
In this article, you have learned how to create and set up the Laravel API in order to create a CRUD API. Utilize the Eloquent Model as an ORM to perform the CRUD operations on the database. Test our API using JavaScript. I hope you will enjoy the article.
Source code: https://github.com/stackpuz/Example-CRUD-Laravel-11
Create a CRUD Web App in Minutes: https://stackpuz.com