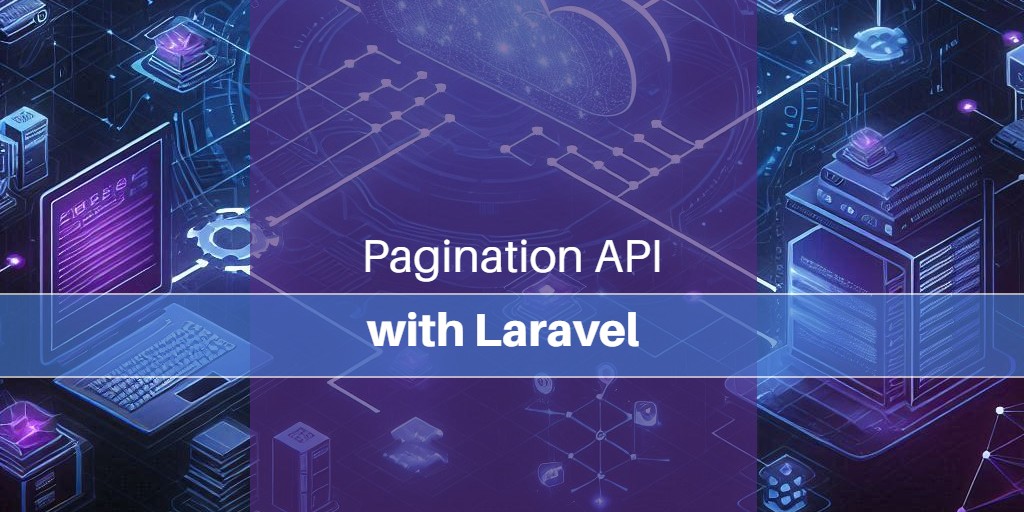
Create a pagination API with Laravel
Splitting larger content into distinct pages is known as pagination. This approach significantly enhances the user experience and speeds up the loading of web pages. This example will demonstrate how to create a pagination API using Laravel and use MySQL as a database.
Prerequisites
- Composer
- PHP 8.2
- MySQL
Setup project
composer create-project laravel/laravel laravel_api 11.0.3
Create a testing database named "example" and run the database.sql file to import the table and data.
Project structure
├─ .env
├─ app
│ ├─ Http
│ │ └─ Controllers
│ │ └─ ProductController.php
│ └─ Models
│ └─ Product.php
├─ bootstrap
│ └─ app.php
├─ resources
│ └─ views
│ └─ index.php
└─ routes
├─ api.php
└─ web.php
*This project structure will show only files and folders that we intend to create or modify.
Project files
.env
This file is the Laravel configuration file and we use it to keep the database connection information.
DB_CONNECTION=mysql
DB_HOST=localhost
DB_PORT=3306
DB_DATABASE=example
DB_USERNAME=root
DB_PASSWORD=
SESSION_DRIVER=file
We also set SESSION_DRIVER=file
to change the session driver from database to file.
app.php
This file is the Laravel application configuration file, and we only added the API routing file here.
<?php
use Illuminate\Foundation\Application;
use Illuminate\Foundation\Configuration\Exceptions;
use Illuminate\Foundation\Configuration\Middleware;
return Application::configure(basePath: dirname(__DIR__))
->withRouting(
web: __DIR__.'/../routes/web.php',
api: __DIR__.'/../routes/api.php',
commands: __DIR__.'/../routes/console.php',
health: '/up',
)
->withMiddleware(function (Middleware $middleware) {
//
})
->withExceptions(function (Exceptions $exceptions) {
//
})->create();
web.php
This file defines the route URL for the Laravel web application. We just changed the default file from welcome.php to index.php.
<?php
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return view('index');
});
api.php
This file defines the route URL for the Laravel API. We define our API route here.
<?php
use App\Http\Controllers\ProductController;
Route::get('/products', [ ProductController::class, 'index' ]);
Product.php
This file defines the Eloquent Model information that maps to our database table named "Product".
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
protected $table = 'Product';
protected $primaryKey = 'id';
}
*To keep the code simple, we define only a few pieces of information here. This is enough for our API.
ProductController.php
This file is used to handle incoming requests and produce the paginated data for the client.
<?php
namespace App\Http\Controllers;
use App\Models\Product;
class ProductController {
public function index()
{
$size = request()->input('size') ?? 10;
$order = request()->input('order') ?? 'id';
$direction = request()->input('direction') ?? 'asc';
$query = Product::query()
->select('id', 'name', 'price')
->orderBy($order, $direction);
$products = $query->paginate($size);
return $products->items();
}
}
We utilize the query string to get $size, $order, $direction
information and create the paginated data by using the paginate($size)
method.
index.php
Instead of entering the URL manually to test our API, we used this file to create links for easier testing.
<!DOCTYPE html>
<head>
</head>
<body>
<ul>
<li><a target="_blank" href="/api/products">Default</a></li>
<li><a target="_blank" href="/api/products?page=2">Page 2</a></li>
<li><a target="_blank" href="/api/products?page=2&size=25">Page 2 and Size 25</a></li>
<li><a target="_blank" href="/api/products?page=2&size=25&order=name">Page 2 and Size 25 and Order by name</a></li>
<li><a target="_blank" href="/api/products?page=2&size=25&order=name&direction=desc">Page 2 and Size 25 and Order by name descending</a></li>
</ul>
</body>
</html>
Run project
php artisan serve
Open the web browser and goto http://localhost:8000
You will find this test page.
Testing
Testing without any parameters
Click the "Default" link, and it will open the URL http://localhost:8000/api/products
The API will return paginated data with default parameters (page = 1 and size = 10).
Page index test
Click the "Page 2" link, and it will open the URL http://localhost:8000/api/products?page=2
The API will return paginated data on the second page, starting with product id 11
Page size test
Click the "Page 2 and Size 25" link, and it will open the URL http://localhost:8000/api/products?page=2&size=25
The API will return paginated data on the second page by starting with product id 26 because the page size is 25.
Order test
Click the "Page 2 and Size 25 and Order by name" link, and it will open the URL http://localhost:8000/api/products?page=2&size=25&order=name
The API will return paginated data on the second page, but the product order is based on the product name.
Descending order test
Click the "Page 2 and Size 25 and Order by name descending" link, and it will open the URL http://localhost:8000/api/products?page=2&size=25&order=name&direction=desc
The API will return paginated data on the second page, but the product order is based on the product name in descending order.
Conclusion
In this article, you have learned how to create and setup the Laravel application in order to implement the pagination API. The pagination approach will enhance the user experience and speed up your Laravel API. If you like the article, please share it with your friends.
Source code: https://github.com/stackpuz/Example-Pagination-Laravel-11
Create a CRUD Web App in Minutes: https://stackpuz.com