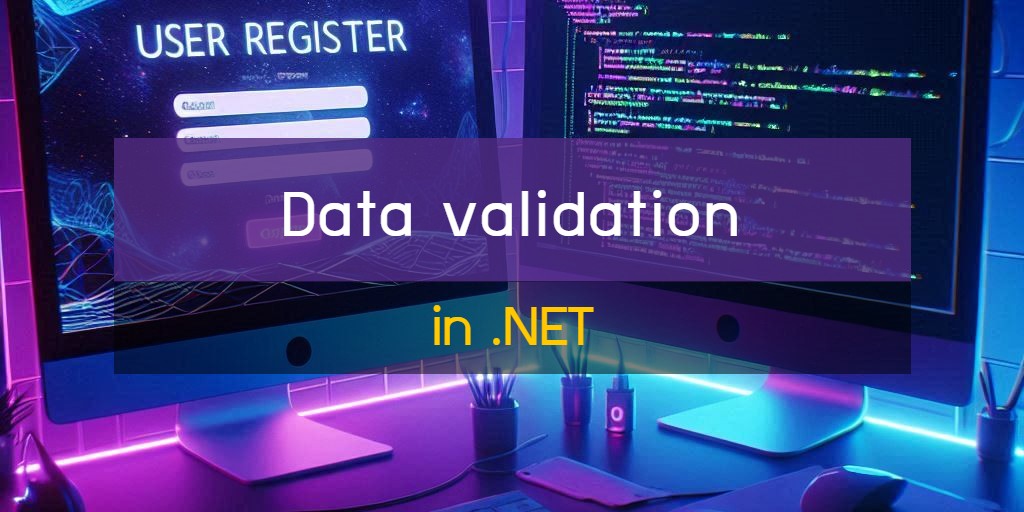
Implement data validation in .NET
Data validation is a crucial aspect of software development, ensuring that input data is accurate and meets required criteria before processing or storage. In .NET, implementing data validation is both straightforward and flexible.
This guide will introduce you to the key concepts and practices for implementing effective data validation in .NET, helping you build secure and reliable applications. From using built-in validation attributes to creating validation logic.
Prerequisites
- .NET 8
Setup project
dotnet new webapi -o dotnet_api -n App
Project structure
├─ App.csproj
├─ Program.cs
├─ Controllers
│ └─ UserController.cs
├─ Models
│ └─ User.cs
└─ Views
└─ User
└─ Create.cshtml
Project files
App.csproj
This file is the .NET project configuration file. We don't use any additional packages for this project.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net8.0</TargetFramework>
<ImplicitUsings>enable</ImplicitUsings>
</PropertyGroup>
</Project>
Program.cs
This file sets up a minimal .NET Core web application. It creates a builder, configures services for MVC with controllers and views.
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddControllersWithViews();
var app = builder.Build();
app.UseRouting();
app.MapControllers();
app.Run();
User.cs
The User class is used for testing validation in the application. It includes data annotations to enforce validation rules:
using System.ComponentModel.DataAnnotations;
namespace App.Models
{
public class User
{
[Required]
public int? Id { get; set; }
[MaxLength(10)]
public string Name { get; set; }
[RegularExpression(@"^\S+@\S+\.\S+$", ErrorMessage="Invalid email address")]
public string Email { get; set; }
[Range(1, 100)]
public int? Age { get; set; }
public DateTime? BirthDate { get; set; }
}
}
Id
Required fieldName
Maximum length of 10 characters.Email
Must match a basic email format.Age
Must be within the range of 1 to 100.BirthDate
Must match a date format.
UserController.cs
This file handles incoming requests and validates user input data.
using Microsoft.AspNetCore.Mvc;
namespace App.Controllers
{
public class UserController : Controller
{
[HttpGet("")]
public IActionResult Create()
{
return View();
}
[HttpPost("submit")]
public IActionResult Submit([Bind]Models.User model)
{
if (ModelState.IsValid)
{
ViewData["pass"] = true;
}
return View("Create", model);
}
}
}
Create()
returns the Create view, which typically contains a form for user input.Submit(..)
processes form submissions and usesModelState.IsValid
to indicate whether the input data is valid. If there are validation errors, the model is returned to display feedback to the user.
Create.cshtml
The HTML user input form is designed to test the validation rules applied to the User model. It typically includes fields that correspond to the properties of the User class.
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
@model App.Models.User
@{
var user = Model;
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<link href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.3.3/css/bootstrap.min.css" rel="stylesheet">
<script>
function fill(valid) {
document.getElementById('id').value = (valid ? '1' : '')
document.getElementById('name').value = (valid ? 'foo' : 'my name is foo')
document.getElementById('email').value = (valid ? 'foo@mail.com' : 'mail')
document.getElementById('age').value = (valid ? '10' : '101')
document.getElementById('birthdate').value = (valid ? '01/01/2000' : '01012000')
}
</script>
</head>
<body>
<div class="container">
<div class="row mt-3">
<form method="post" action="submit">
<div class="mb-3 col-12">
<label class="form-label" for="id">Id</label>
<input id="id" name="Id" class="form-control form-control-sm" asp-for="@user.Id" />
<span asp-validation-for="Id" class="text-danger"></span>
</div>
<div class="mb-3 col-12">
<label class="form-label" for="name">Name</label>
<input id="name" name="Name" class="form-control form-control-sm" asp-for="@user.Name" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="mb-3 col-12">
<label class="form-label" for="email">Email</label>
<input id="email" name="Email" class="form-control form-control-sm" asp-for="@user.Email" />
<span asp-validation-for="Email" class="text-danger"></span>
</div>
<div class="mb-3 col-12">
<label class="form-label" for="age">Age</label>
<input id="age" name="Age" class="form-control form-control-sm" asp-for="@user.Age" />
<span asp-validation-for="Age" class="text-danger"></span>
</div>
<div class="mb-3 col-12">
<label class="form-label" for="birthdate">Birth Date</label>
<input id="birthdate" name="BirthDate" class="form-control form-control-sm" asp-for="@user.BirthDate" type="text" />
<span asp-validation-for="BirthDate" class="text-danger"></span>
</div>
<div class="col-12">
<input type="button" class="btn btn-sm btn-danger" onclick="fill(0)" value="Fill invaid data" />
<input type="button" class="btn btn-sm btn-success" onclick="fill(1)" value="Fill vaid data" />
<button class="btn btn-sm btn-primary">Submit</button>
</div>
@if (ViewData["pass"] != null) {
<div class="alert alert-success mt-3">
Validation success!
</div>
}
</form>
</div>
</div>
</body>
asp-validation-for
displays validation errors for a specific property of the model.ViewData["pass"]
indicates whether the input data is valid and can be used to show a validation success message.
Run project
dotnet run
Open the web browser and goto http://localhost:5122
You will find this test page.
Testing
Click "Fill invalid data" and then click "Submit" to see the error messages displayed in the input form.
Click "Fill valid data" and then "Submit" again. You should see the validation success message displayed in the input form.
Conclusion
This article has covered implementing validation from models to views, helping you build reliable and user-friendly applications. Apply these practices to enhance both the robustness and usability of your .NET projects.
Source code: https://github.com/stackpuz/Example-Validation-dotnet-8
Create a CRUD Web App in Minutes: https://stackpuz.com