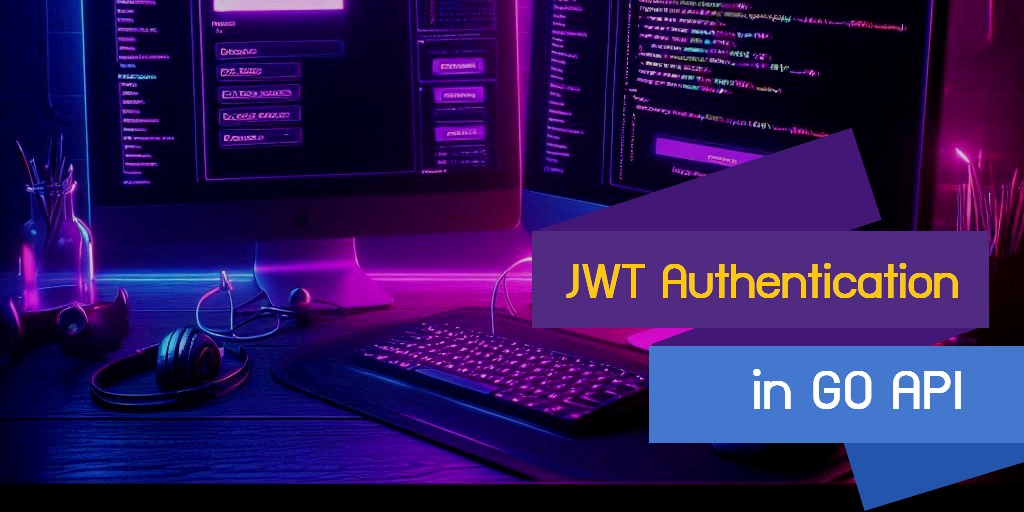
Implementing JWT Authentication in Go API
JWT (JSON Web Token) is a highly effective method for securing APIs through token-based authentication, ensuring that only authenticated users can access your API endpoints. Unlike traditional session-based approaches, JWT is stateless, eliminating the need for server-side session storage, which makes it ideal for scalable and performant applications. In this guide, we'll walk you through implementing JWT authentication in a Go API, from generating tokens upon user login to securing your endpoints by validating these tokens, ultimately enhancing the security and robustness of your application's data and resources.
Prerequisites
- Go 1.21
Setup project
go mod init app
go get github.com/gin-gonic/gin@v1.5.0
go get github.com/golang-jwt/jwt
go get github.com/joho/godotenv
Project structure
├─ .env
├─ main.go
├─ middleware
│ └─ authenticate.go
└─ public
├─ index.html
└─ login.html
Project files
.env
jwt_secret = b0WciedNJvFCqFRbB2A1QhZoCDnutAOen5g1FEDO0HsLTwGINp04GXh2OXVpTqQL
This .env
file contains a single environment variable jwt_secret
, which holds a secret key used for signing and verifying JWT tokens in the application.
authenticate.go
package middleware
import (
"net/http"
"os"
"strings"
"github.com/gin-gonic/gin"
"github.com/golang-jwt/jwt"
)
type Claims struct {
Id int `json:"id"`
Name string `json:"name"`
jwt.StandardClaims
}
func Authenticate() gin.HandlerFunc {
return func(c *gin.Context) {
if c.Request.URL.Path == "/" || c.Request.URL.Path == "/login" {
c.Next()
return
}
authHeader := c.GetHeader("Authorization")
if authHeader == "" {
c.Status(http.StatusUnauthorized)
c.Abort()
return
}
tokenString := strings.TrimPrefix(authHeader, "Bearer ")
token, err := jwt.ParseWithClaims(tokenString, &Claims{}, func(token *jwt.Token) (interface{}, error) {
return []byte(os.Getenv("jwt_secret")), nil
})
if err != nil || !token.Valid {
c.Status(http.StatusUnauthorized)
c.Abort()
return
}
if claims, ok := token.Claims.(*Claims); ok {
c.Set("user", claims)
} else {
c.Status(http.StatusUnauthorized)
c.Abort()
return
}
c.Next()
}
}
The authenticate.go
middleware defines a function for JWT authentication in a Go API using the Gin framework. It checks if the request is for the /
or /login
paths, in which case no authentication is needed. For other routes, it retrieves the Authorization
header, expecting a Bearer token. The token is parsed and validated using the jwt
package and a secret key from environment variables. If the token is invalid or missing, the request is aborted with a 401 Unauthorized
status. If valid, the user claims (such as id
and name
) are extracted and added to the Gin context, allowing access to protected routes.
main.go
package main
import (
"app/middleware"
"net/http"
"os"
"time"
"github.com/gin-gonic/gin"
"github.com/golang-jwt/jwt"
"github.com/joho/godotenv"
)
func main() {
godotenv.Load()
router := gin.Default()
router.Use(middleware.Authenticate())
router.LoadHTMLFiles("public/index.html", "public/login.html")
router.GET("/", func(c *gin.Context) {
c.HTML(http.StatusOK, "index.html", nil)
})
router.GET("/login", func(c *gin.Context) {
c.HTML(http.StatusOK, "login.html", nil)
})
router.GET("/user", func(c *gin.Context) {
user, _ := c.Get("user")
claims := user.(*middleware.Claims)
c.JSON(http.StatusOK, gin.H{"name": claims.Name})
})
router.POST("/login", func(c *gin.Context) {
var login map[string]string
c.BindJSON(&login)
if login["name"] == "admin" && login["password"] == "1234" {
token := jwt.NewWithClaims(jwt.SigningMethodHS256, &middleware.Claims{
Id: 1,
Name: login["name"],
StandardClaims: jwt.StandardClaims{
IssuedAt: time.Now().Unix(),
ExpiresAt: time.Now().Add(24 * time.Hour).Unix(),
},
})
tokenString, _ := token.SignedString([]byte(os.Getenv("jwt_secret")))
c.JSON(http.StatusOK, gin.H{"token": tokenString})
} else {
c.Status(http.StatusBadRequest)
}
})
router.Run()
}
The main.go
file sets up a Go web server using the Gin framework to handle routes with JWT-based authentication. It uses middleware for authentication, which checks for valid JWT tokens in requests. The server serves two HTML pages: index.html
and login.html
, which are accessible via the /
and /login
routes.
For the /user
route, the server retrieves the authenticated user's name from the JWT claims and returns it in the response. For the /login
POST route, the server validates user credentials (name
and password
) and, if valid, generates a JWT token, signing it with a secret key and sending it back to the client. The server is configured to listen for requests and run on the default port.
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<link href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.3.3/css/bootstrap.min.css" rel="stylesheet">
<link href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.5.0/css/all.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="row">
<div class="col mt-5">
<div class="d-flex justify-content-center">
<div class="card p-0">
<div class="card-header">
<h3>Home</h3>
</div>
<div class="card-body text-center">
<i id="icon" class="fa fa-times-circle fa-5x mt-3 text-danger"></i>
<p id="message" class="mt-3">
You are currently not logged in.
<br/>
Redirecting to login page in seconds..
</p>
<div id="logout" class="col-12 d-none">
<button class="btn btn-primary w-100" onclick="logout()">Logout</button>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<script>
function init() {
fetch('/user', {
headers: {
Authorization: 'Bearer ' + localStorage.getItem('token')
}
}).then(response => {
if (response.ok) {
response.json().then(data => {
document.getElementById('icon').className = 'fa fa-check-circle fa-5x mt-3 text-success'
document.getElementById('message').innerText = 'You are currently logged in as: ' + data.name
document.getElementById('logout').classList.remove('d-none')
})
}
else {
if (response.status === 401) {
setTimeout(() => {
location = '/login'
}, 4000)
}
else {
alert(`Error: ${response.status} ${response.statusText}`)
}
}
})
}
function logout() {
localStorage.removeItem('token')
location.reload()
}
init()
</script>
</body>
The index.html
is a simple web page that provides a user interface for displaying the login status of a user. It uses Bootstrap for styling and Font Awesome for icons. On page load, it checks the user's authentication status by sending a request to the server with a JWT token stored in localStorage
. If the user is logged in, it shows a success message with the user's name and a logout button. If not logged in, it shows a message indicating the user is not logged in and redirects them to the login page after a few seconds.
login.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<link href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.3.3/css/bootstrap.min.css" rel="stylesheet">
<link href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.5.0/css/all.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="row">
<div class="col mt-5">
<div class="d-flex justify-content-center">
<div class="card p-0" style="width: 300px;">
<div class="card-header">
<h3>Login</h3>
</div>
<div class="card-body">
<i class="fa fa-user-circle fa-5x d-block text-center text-secondary"></i>
<form onsubmit="return login()">
<div class="row">
<div class="mb-3 col-12">
<label class="form-label" for="user_account_name">User Name</label>
<input id="name" class="form-control form-control" value="admin" required/>
</div>
<div class="mb-3 col-12">
<label class="form-label" for="user_account_password">Password</label>
<input id="password" class="form-control form-control" type="password" value="1234" required/>
</div>
<div class="col-12">
<button class="btn btn-primary w-100">Login</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</div>
<script>
function login() {
fetch('/login', {
method: 'POST',
body: JSON.stringify({
name: document.getElementById('name').value,
password: document.getElementById('password').value
}),
headers: {
'Content-Type': 'application/json'
}
}).then(response => {
if (response.ok) {
response.json().then(data => {
localStorage.setItem('token', data.token)
location = '/'
})
}
else {
alert(`Error: ${response.status} ${response.statusText}`)
}
})
return false
}
</script>
</body>
The login.html
page provides a simple login form where users can input their username and password. It uses Bootstrap for styling and Font Awesome for icons. When the user submits the form, a JavaScript function login()
sends a POST
request to the /login
endpoint with the entered credentials. If the login is successful, the server returns a JWT token, which is stored in localStorage
. The page then redirects the user to the home page (/
). If the login fails, an error message is displayed.
Run project
go run main.go
Open the web browser and goto http://localhost:8080
You will find this test page.
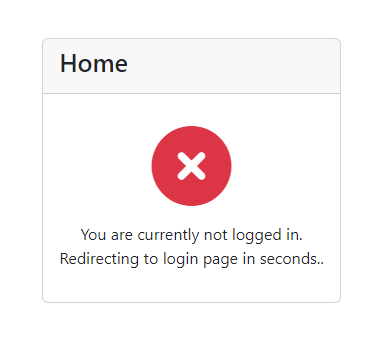
Testing
After a few seconds, you will be redirected to the login page.
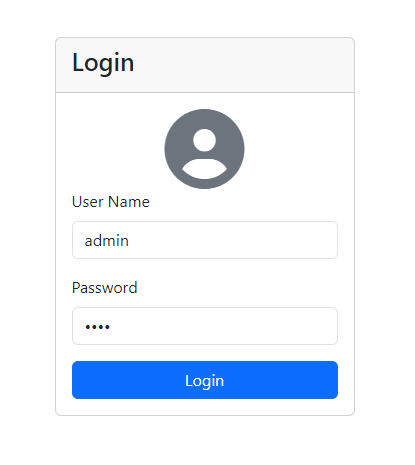
Press the login button, and you will be logged in to the home page, which will display the logged-in user's name.
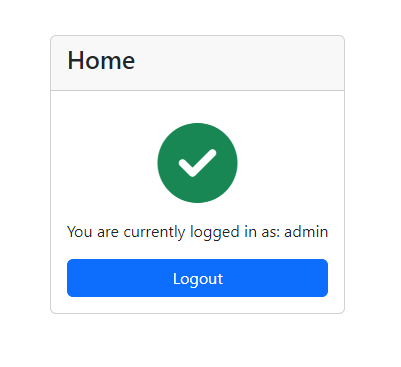
Try refreshing the browser, and you will see that you're still logged in. Then, press the logout button, the JWT token will be removed, and you will be redirected to the login page again.
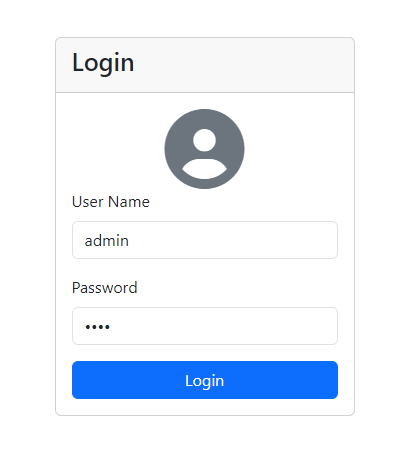
Conclusion
In conclusion, implementing JWT authentication in a Go API provides a secure and scalable approach to handle user authentication. By using the Gin framework alongside the golang-jwt/jwt
package, we can easily integrate token-based authentication into our application. JWT tokens are generated during login, securely validating user credentials, and granting access to protected routes. The middleware ensures that only authenticated users can access these routes by verifying the token’s validity. This stateless authentication mechanism offers enhanced performance and flexibility, making it an ideal choice for modern API architectures.
Source code: https://github.com/stackpuz/Example-JWT-Go
Create a CRUD Web App in Minutes: https://stackpuz.com